TransformPoint Control
User manual of the trinckle paramate GUI Controls API.
Introduction
The TransformPoint control is a versatile component within the paramate GUI Controls API that allows for the selection, movement, and placement of geometry in a 3D environment. This control offers three primary use-cases, providing flexible functionality for interacting with geometry without the need for ray probing.
1. Selecting and Deselecting Geometry for Manipulation
One of the key functionalities of the TransformPoint control is its ability to select and deselect geometry for manipulation. By attaching the TransformPoint control to the specific geometry, a user can mark that geometry as selected. This selection enables the user to perform various operations on the geometry, such as applying modifiers, aligning it with other selected geometry, or triggering specific actions related to the selected geometry.
This feature enhances the configurator experience by allowing users to easily interact with and modify specific elements within the 3D scene. By selecting geometry using the TransformPoint control, users can effectively isolate and manipulate individual objects or groups of objects for customization and adjustment.
2. Moving Geometry by Clicking and Dragging
The TransformPoint control provides intuitive functionality for moving geometry within the 3D space. By clicking and dragging the TransformPoint control attached to the geometry, users can effortlessly change its position on the screen. This feature enables assisted placement and fine-tuning of the geometry's location.
Whether it's repositioning an existing object or adjusting the placement of newly added geometry, the TransformPoint control streamlines the process of moving objects within the 3D environment. Users can easily align and position geometry exactly where they want it, ensuring accurate spatial arrangements.
3. Adding and Deleting Geometry
The TransformPoint control also supports the addition and deletion of geometry elements. By configuring the control appropriately, users can leverage its functionality to simplify the process of adding new geometry or deleting existing geometry.
To add new geometry, users can click on the screen using the TransformPoint control, defining the desired position for the new element. This feature facilitates the easy placement of geometry at specific locations, enabling users to dynamically expand their scene with additional objects.
Conversely, users can select the TransformPoint control attached to existing geometry to initiate deletion. This functionality allows for the straightforward removal of selected geometry, streamlining the editing and refinement process.
Control Initialization
The TransformPoint control can be initialized using the TransformPoint constructor function. This function allows the initialization of TransformPoint control with or without being bound to a paramate open parameter. When a paramate open parameter is specified, the control establishes a direct connection with the paramate server and enables real-time updates and interactions. For more information about these two types of initialization, see also GUI Controls Initialization.
The options object provides settings for customizing the behavior of the transformPoint control. These settings include following optional configuration parameters: dragModeActive, connectLines, drawLabel, and labelText.
For the explanation of the options object's settings please consult TransformPoint constructor function.
Below is the example that initializes TransformPoint with default settings (e.g., custom settings are undefined) and with open parameter string.
Example
const transformPoint = new ParamateControls.TransformPoint(viewport, controls, undefined, 'main:position');
In trCAD, open parameter can be defined like this:
Example
open vector position
{
name = "TransformPoint position"
descr = "Position vector for TransformPoint control"
value = <[10, 10, 10]>
}
get (position)
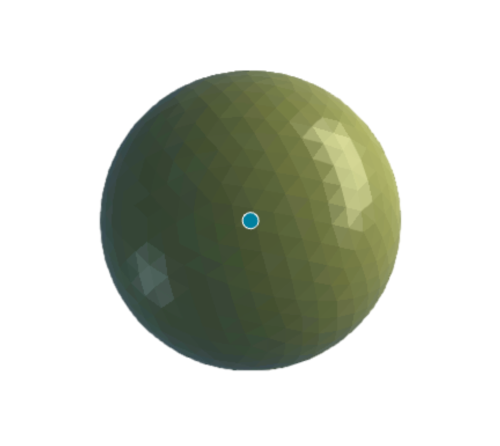
Single TransformPoint instance with default settings. The open parameter is defined as a single vector.
In the example below, the TransformPoint is initialized with custom options, where the connectLines parameter is set to true. In addition, the open parameter string is defined as a list of vectors to create a list of points:
Example
const transformPoints = new ParamateControls.TransformPoint(viewport, controls, { connectLines: true }, 'main:positionList');
In trCAD, open parameter would look like this:
Example
open vector positionList[]
{
name = "TransformPoint position list"
descr = "List of position vectors for TransformPoint control"
value = [<[0, 0, 0]>,<[10, 5, 0]>,<[20, 1, 0]>]
}
get (positionList)
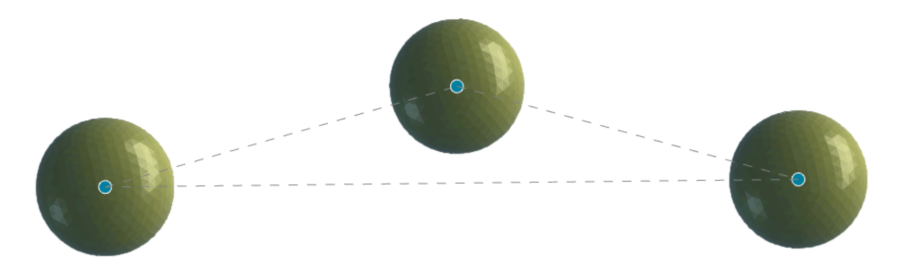
Single TransformPoint instance with custom settings (e.g., connected lines are drawn). The open parameter is defined as a list of vectors.
Look and Feel Customization
To modify the various visual aspects of the controls, such as fillStyle, strokeStyle, fillText, font, lineWidth, and more, users can create custom styling presets using the following setters:
Each styling setter setups a list of styling presets confirming to the corresponding interface.
For example, to create a preset of styles for the point that would vary point radius, fill- and stroke color, line type and thickness (in case the points should be connected with the line), a user may create a following pointStyles setting object:
Example
// Initialize TransformPoint object.
const transformPoints = new ParamateControls.TransformPoint(viewport, controls, { connectLines: true }, 'main:positionList');
// Initialize a preset of 2 styles for the point
const pointStyle = [
{
radius: 5,
strokeStyle: '#D1E8E2',
fillStyle: '#3D405B',
lineWidth: 1,
lineDash: []
},
{
radius: 7,
strokeStyle: '#E2E2E2',
fillStyle: '#E07A5F',
lineWidth: 1,
lineDash: []
}
];
transformPoints.pointStyles = pointStyles;
While pointStyles[0] styles a point in its non-selected state, pointStyles[1] styles a point in the selected state.
Next, to change a style for connecting line, the preset may look like this:
Example
// Initialize a preset of 1 style for a point
const lineStyles = [
{
strokeStyle: '#81B29A',
lineWidth: 1,
lineDash: [5, 5],
},
];
transformPoints.lineStyles = lineStyles;
The styling above will produce the following result:
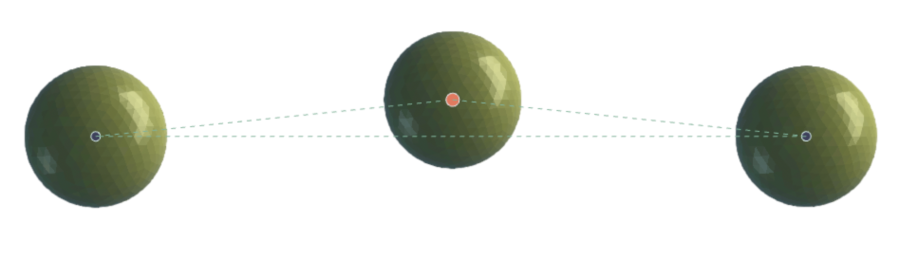
TransformPoint control with the styling applied to points. While middle point is in selected state (pointStyles[1]), other points are in non-selected state (pointStyles[0]).
Internal
TODO: explain that presets can be generic or customised (maybe review how presets are setup right now)
Default Key Events
Event | Key | Behaviour |
keydown | 'H' or 'h' | show / hide help window |
keydown | ctrlKey or metaKey (on Mac OS) | select multiple points |