GUI Controls User Events
User manual of the trinckle paramate GUI Controls API.
The paramate GUI controls library allows to handle various process events. The described below events reflect steps in the given process chain during the setup and usage of the control elements. Users of the library can define their own callback functions that are called when the respective event occurs.
This page gives a general overview over the most important aspects of this topic:
- List of Available Events
- Setting Up of Event Callbacks
- Return Values
- Sequence of Events
- Sequence "Mouse Manipulation"
- Sequence "Configuration"
List of Available Events
The events available for the GUI controls are:
Event Name | Description | Returns |
onMouseMainDown | Function that is called when a user presses the main mouse button over a control element. | bool |
onMouseMainMove | Function that is called when the pointer is moving while the main mouse button is pressed over a control element. | none |
onMouseMainUp | Function that is called when a user releases the main button over a control element. | none |
onConfigure | Function that is called when the control parameter values got updated by the system. This happens in two different cases: either during initialization of the configurator or after each subsequent configuration of the model (see sketch below). |
none |
onShow | Function that is called when the control is being displayed. | none |
onHide | Function that is called when the control is being hidden. | none |
onValueChange | Function that is called when the value of the configurator is being changed. | bool |
All events of the base JS API can be used in combination with those of the GUI controls.
Certain methods allow the setting of additional callback functions whose conditions for execution are explained in the documentation of the respective methods.
Setting Up of Event Callbacks
Event callback functions can be set up during the session by method setCallbacks.
Example
// Assuming, there is an instance of a TransformPoint control,
// initialize the callbacks
const onMouseMainDown = () => {
console.log('Calling custom callback on mouse down event');
return true;
};
const onMouseMainUp = () => {
console.log('Calling custom callback on mouse up event');
};
const onConfigure = () => {
console.log('Calling custom callback on configure');
};
// Save callbacks in the object for convenience
const cbOptions = {
onMouseMainDown,
onMouseMainUp,
onConfigure
};
// Set the callbacks by calling setCallbacks function
// on an instance of TransformPoint control
transformPoint.setCallbacks(cbOptions);
Method clearCallbacks can be used to unset all event callback functions again.
Return Values
Some callback functions should return a boolean value true or false. These are functions that are called synchronously at a certain point of a process chain. Based on the actual state of the process, the function implementation can decide whether the process chain shall proceed or whether it should be terminated at this point. To proceed, the callback function must return a value of true. If it, on the other hand, returns false, the process chain will be stopped at this point.
Sequence of Events
In the following, some processes are explained with the help of sequence diagrams. This is a suitable way to depict the interactions between users and various code modules ("actors") along the flow of time (vertical axis, from top to bottom). Arrows with solid heads represent synchronous requests or function calls in which the calling actor does not proceed its execution until the request or function returns. Arrows with hollow line heads are asynchronous calls in which the calling code keeps on executing. Dashed arrows are returned values of the called functions or requests. Note that, by nature of asynchronicity, multiple functions that are asynchronously called in short sequence are not guaranteed to execute one after the other, even if this might be implied by their order in the diagrams.
The sequence diagrams do not contain those additional callback functions that can be set as argument of certain special library methods.
Internal
TODO: Maybe all of these methods could be removed from the public documentation; then this note and the corresponding one aboove could be erased.
Sequence "Mouse Manipulation"
Many GUI controls contain elements that can be manipulated by mouse action. Some events are available to control this behavior.
Internal
TODO: We need to add touch action as well.
The events for the main mouse button are called on pressing and releasing the button over the GUI control or on moving the mouse while the button is pressed. Some events expect a return value true in order to proceed, otherwise the interaction is canceled. The following diagram shows the process:
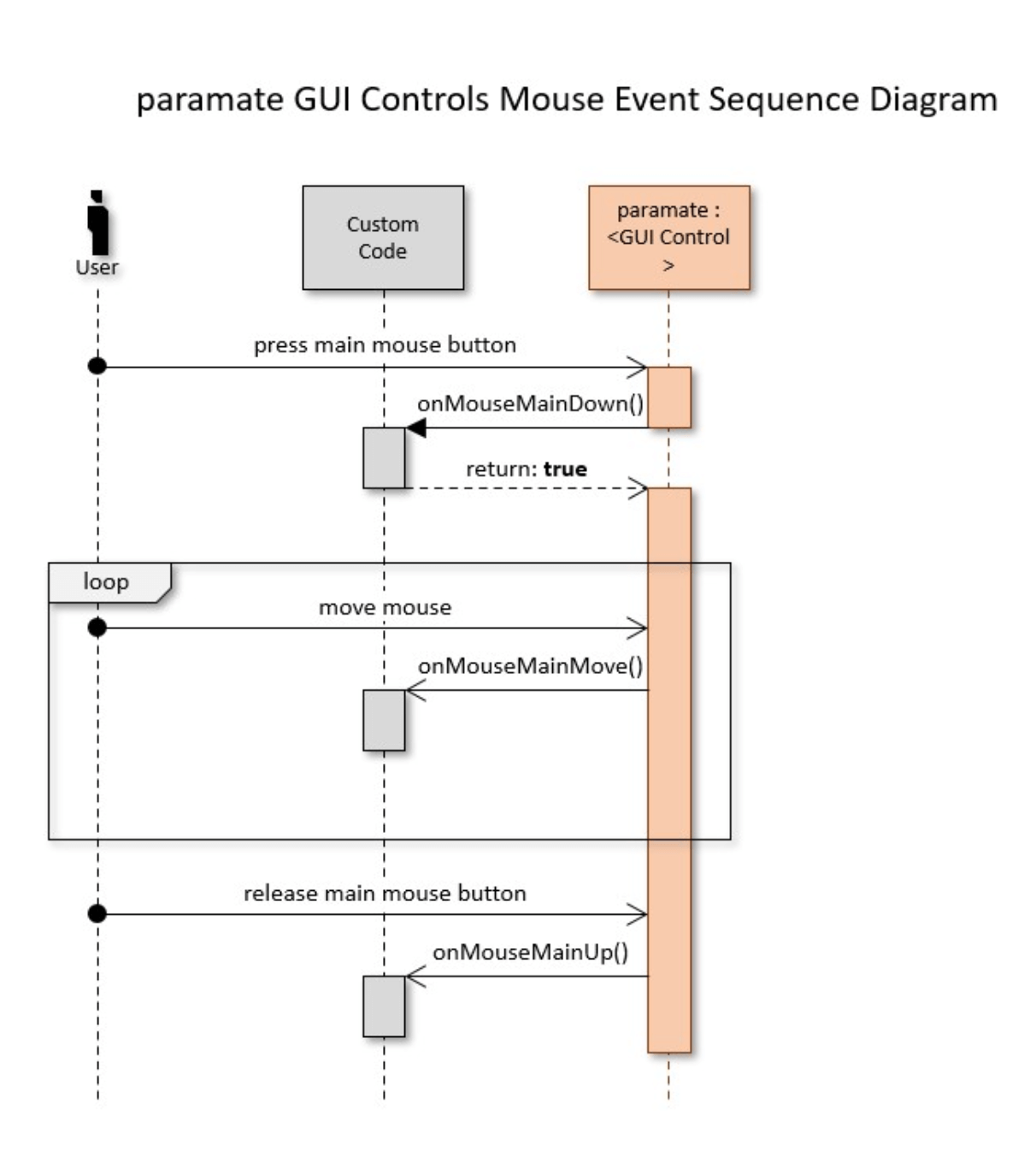
Sequence diagram of a mouse main button click on the GUI control with subsequent movement. Note that the onMouseMainMove event is repeatedly called whenever the mouse is moved during the process.
Sequence "Configuration"
The following sequence diagram shows the process of one model configuraton, i.e. the chain of events that is triggered when the user applies a change to an GUI control element. It shows the most important internal interactions between the paramate cloud server, the configurator and viewport elements of the paramate JavaScript base API, the GUI controls module and the custom code, i.e. the user-defined callback functions. The callbacks in the diagram also include events of the base JS API.
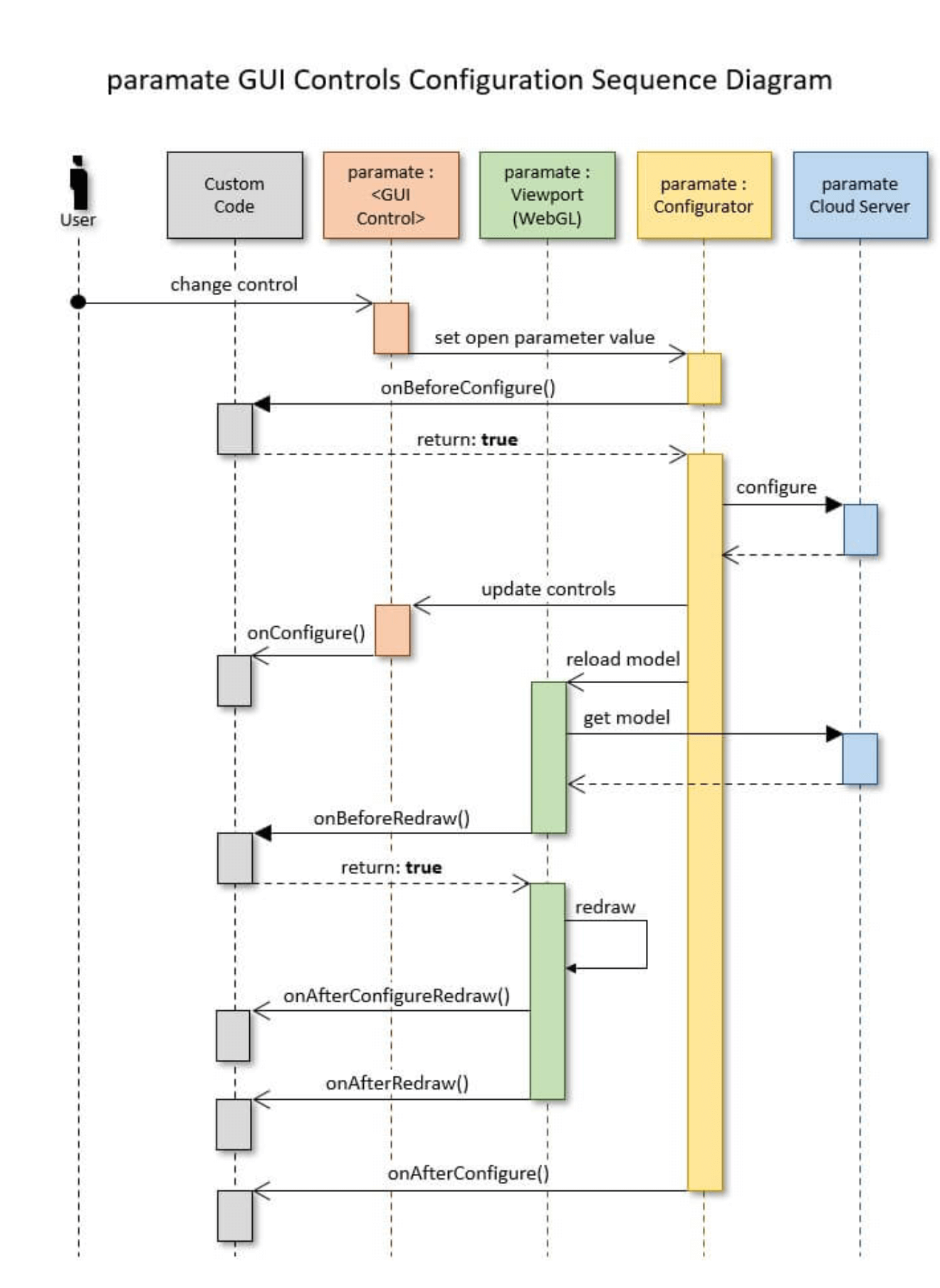
Sequence diagram of a single configuration process. This is repeated each time the value of a control gets modified by the user. The various custom events are denoted. (Not included are additional callbacks that are given as arguments of different functions.)